jPDFSecure Developer Guide
jPDFSecure Developer Guide
Contents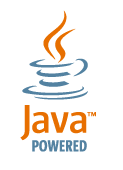
Introduction
Getting Started
Digitally Signing a PDF document
Getting Current Password / Encryption Info
Getting Current Permissions
Changing Password Permissions
Clearing Password Permissions
Getting Basic Document Information
Distribution and JAR files
Javadoc API
Source Code Samples
Introduction
jPDFSecure is a Java library that integrates seamlessly into your application to update security settings of your PDF documents. jPDFSecure provides the following functions:
- Sign PDF documents
- Encrypt / Decrypt PDF documents
- Set / Remove Permissions
- Permission to Print At High Resolution
- Permission to Print
- Permission to Copy or Extract Content
- Permission to Extract Content in Support of Accessibility for Disabled Users
- Permission to Modify
- Permission to Assemble: Insert, Rotate, Delete Pages
- Permission to Add / Modify Annotations
- Permissions to Fill Form Fields and Sign
- Set / Remove Passwords
- Password to Open PDF Documents
- Master Password to Change Permissions
Like all of our libraries, jPDFSecure is built on top of Qoppa’s proprietary format and doesn’t require any third party programs or drivers.
Getting Started
The starting point for using jPDFSecure is the com.qoppa.pdfSecure.PDFSecure. This class is used to load a pdf document and to update security settings of the pdf document. The class provides three constructors to load PDF files from the file system, a URL or an InputStream. All constructors take an additional parameter, an object that implements IPasswordHandler, that will be queried if the PDF file requires a password to open (called Open or User password). For PDF files that are not currently encrypted, this second parameter can be null:
PDFSecure pdfSecure = new PDFSecure(new URL("http://www.mysite.com/content.pdf"), null); |
Digitally Signing a PDF document
There are 3 steps to add a new digital signature to a PDF document with jPDFSecure: create a new digital signature field, load digital id from a keystore to create a SignatureInformation object and apply the signature:
// Create signature field on the first page Rectangle2D signBounds = new Rectangle2D.Double (36, 36, 144, 48); SignatureField signField = pdfSecure.addSignatureField(0, "signature", signBounds); // Load the keystore that contains the digital id to use in signing FileInputStream pkcs12Stream = new FileInputStream ("https://www.qoppa.com/files/pdfsecure/guide/sourcesamples/keystore.pfx"); KeyStore store = KeyStore.getInstance("PKCS12"); store.load(pkcs12Stream, "store_pwd".toCharArray()); pkcs12Stream.close(); // Create signing information SigningInformation signInfo = new SigningInformation (store, "key_alias", "key_pwd"); // Apply digital signature pdfSecure.signDocument(signField, signInfo); // Save the document pdfSecure.saveDocument ("output.pdf"); |
To sign an existing digital signature field, you would simply replace the first step by:
// Get first signature field SignatureField signField = (SignatureField) pdfSecure.getSignatureFields().get(0); |
Getting Current Password / Encryption Info
Once a PDFSecure object has been created, the host application can call simple method to get current security settings of the loaded PDF document.
// PDF document is encrypted System.out.println(pdfSecure.isEncrypted()); // PDF document has an Open (or User) password (password to open the document) System.out.println(pdfSecure.hasOpenPassword()); // PDF document has a Permissions (or Owner) password (password to update permissions) System.out.println(pdfSecure.hasPermissionsPassword()); |
Getting Current Permissions
PDFSecure.getPDFPermissions will return a permission object. From this object, it is easy to get all the distinct permissions.
AllPDFPermissions allPerms = pdfSecure.getPDFPermissions(); |
AllPDFPermissions encompasses 3 levels of permissions in a PDF document:
- Password Permissions (when the document is encrypted), accessible through perms.getPasswordPermissions()
- DocMDP Permissions (when the document is certified with a certifying signatures), accessible through perms.getDocMDPPermissions()
- Usage Rights Permissions (when the document is Reader enabled), accessible through perms.getDocMDPPermissions()
// permission to print high resolution System.out.println(allPerms.isPrintHighResAllowed()); // permission to print // automatically on if the permission to print high res is on System.out.println(allPerms.isPrintAllowed()); // permission to copy text and graphics System.out.println(allPerms.isExtractTextGraphicsAllowed()); // permission to copy text and graphics for disabled users // automatically on of the permissions to copy text and graphics is on System.out.println(allPerms.isExtractTextGraphicsForAccessibilityAllowed()); // permission to change the document System.out.println(allPerms.isChangeDocumentAllowed()); // permission to assemble document: insert, rotate, delete pages // automatically on if the permission to change document is on System.out.println(allPerms.isAssembleDocumentAllowed()); // permission to add/modify annotations // automatically on if the permission to change document is on System.out.println(allPerms.isModifyAnnotsAllowed()); // permission to fill form fields and sign // automatically on if the permission to change document is on // also automatically on if the permission to modify annotations is on System.out.println(allPerms.isFillFormFieldsAllowed()); |
Changing Password Permissions
PDFSecure.setSecurity is the method to call to encrypt the document and set / update password permissions.
// Create permissions object to allow printing, assembling and filling forms only PasswordPermissions perms = new PasswordPermissions (false); perms.setPrintAllowed(true); perms.setAssembleDocumentAllowed(true); perms.setFillFormsAllowed(true); // Set new password permissions pdfSecure.setPasswordPermissions("owner", "user", perms, currentPermPwd, PasswordPermissions.ENCRYPTION_AES_128); // save pdf document (outputPDFFile is a Java File Object) pdfSecure.saveDocument(outputPDFFile.getAbsoluteFileName()); |
The parameters to the setPasswordPermissions method are:
- newPermissionsPwd – This will become the new permissions password.
- newOpenPwd – This will become the new open password.
- permissions – The PasswordPermissions object.
- currentPermissionsPwd – The current permission password if the PDF document already has a permissions password
- encryptType – The encryption type to use: PasswordPermissions.ENCRYPTION_RC4_128, PasswordPermissions.ENCRYPTION_AES_128, PasswordPermissions.ENCRYPTION_AES_256, etc..
Note that there is some hierarchy in permissions:
- If the permissions to Print High Res is on then the permission to Print is automatically on.
- If the permissions to Extract Text and Graphics is on then the permission to Extract Text and Graphics for Accessibility is automatically on.
- If the permission to Change Document is on then the following 3 permissions are on: Assemble Document, Modify Annotations, Fill Form Fields.
- If the permission to Modify Annotations is on then the permissions to Fill Form Fields is automatically on.
Clearing Password Permissions
Calling PDFSecure.clearPasswordPermissions will clear all password permissions for this document: it will clear open/user password, owner/permissions password, encryption. This means that all password permissions will be reset to true. If the PDF document has a permissions password, it will have to be passed on as a parameter (currentPermissionsPwd below), else it can be left null:
// clear all security settings pdfSecure.clearPasswordPermissions(currentPermissionsPwd); // save pdf document (outputPDFFile is a Java File Object) pdfSecure.saveDocument(outputPDFFile.getAbsoluteFileName()); |
Getting Basic Information about the PDF Document (Title, Author, etc…)
To get basic information about the loaded PDF document, you need to get the DocumentInfo class accessible through PDFSecure.getDocumentInfo. From this class, you can get information about the document such as title, author, subject, keywords, etc…
System.out.println(pdfSecure.getDocumentInfo().getTitle()); System.out.println(pdfSecure.getDocumentInfo().getAuthor()); System.out.println(pdfSecure.getDocumentInfo().getKeywords()); |
Distribution and JAR Files
Required and optional jar files for jPDFSecure can be found on the jPDFSecure Download page.